A video encoder is a software or hardware application that compresses video files. The goal of video encoding is to reduce the size of a video file without sacrificing too much quality. This is done by removing redundant data from the video file.
In PHP, you can use the FFmpeg library to encode and manipulate videos. FFmpeg is a powerful multimedia framework that allows you to work with audio and video files, including encoding, decoding, transcoding, and much more. To use FFmpeg in PHP, you’ll typically execute FFmpeg commands using the exec()
or shell_exec()
functions. Before proceeding, make sure that FFmpeg is installed on your server.
Here’s a basic example of how you can use FFmpeg in PHP to encode a video:
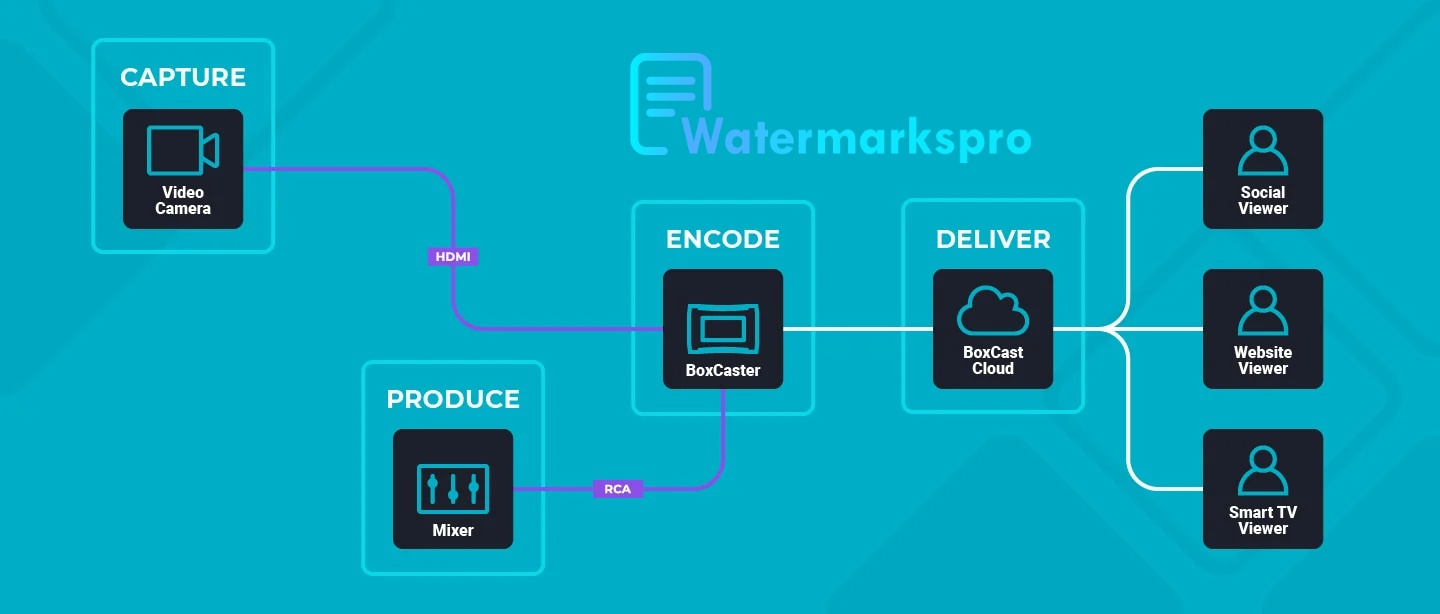
<?php
// Input video file path
$inputFile = ‘/path/to/input_video.mp4’;
// Output video file path
$outputFile = ‘/path/to/output_video_encoded.mp4’;
// Set the desired video bitrate (in kbps)
$bitrate = ‘2000’;
// FFmpeg command to encode the video with the desired bitrate
$ffmpegCommand = “ffmpeg -i $inputFile -b:v {$bitrate}k $outputFile 2>&1”;
// Execute the FFmpeg command
exec($ffmpegCommand, $output, $returnCode);
// Check if FFmpeg command was successful
if ($returnCode === 0) {
echo ‘Video encoding successful!’;
} else {
echo ‘Video encoding failed. Error message: ‘ . implode(“\n”, $output);
}
?>
In this example, we use the -b:v
option to set the video bitrate, which determines the video’s quality and file size. You can adjust the bitrate
value to suit your specific requirements.
Please note that using exec()
or shell_exec()
to run external commands can have security implications. Make sure to validate user inputs and sanitize data to prevent any potential security vulnerabilities.
There are many different video encoders available, each with its own strengths and weaknesses. Some of the most common video encoders include:
- H.264 (MPEG-4 AVC): This is the most common video encoder today. It offers a good balance of quality and file size, and it is supported by most devices and platforms.
- H.265 (MPEG-H HEVC): This is a newer video encoder that offers even better compression than H.264. However, it is not as widely supported as H.264.
- VP9 (Google): This is a newer video encoder that is gaining popularity. It offers good compression and is supported by most modern web browsers.
The video encoding process typically involves the following steps:
- The video file is broken down into individual frames.
- Each frame is analyzed to determine which parts of the frame are important and which parts can be discarded.
- The important parts of the frame are encoded using a compression algorithm.
- The encoded frames are then reassembled into a new video file.
The amount of compression that is applied to a video file depends on a number of factors, including the desired file size, the quality of the video, and the capabilities of the encoder.
Here are some of the factors that affect the quality of a video after encoding:
- The video encoder: Some video encoders are better at preserving quality than others.
- The compression settings: The compression settings can be adjusted to trade off between quality and file size.
- The video content: Some video content is more difficult to compress than others.
The quality of a video after encoding can be subjectively assessed by watching the video. However, there are also some objective measures of video quality, such as the PSNR (Peak Signal-to-Noise Ratio) and SSIM (Structural Similarity Index).
I hope this helps! Let me know if you have any other questions.